This post is about running an Angular 2 app in Android emulator.
We have seen that the documentation provided on internet is not the way it should be. This is because it includes four entirely different setups (Node, Android SDK, NativeScript and GIT). Issues occurs and we end up in wasting much time in internet search to fix those issue. We know the issues well and hence have tried to document this process from scratch where you have just....
>>>> Windows 10 installed on your machine. .... And Nothing Else
Please follow the mentioned steps in sequence (remember the only pre-requisite being Windows 10).
You can download the same from -
https://nodejs.org/en/download/current
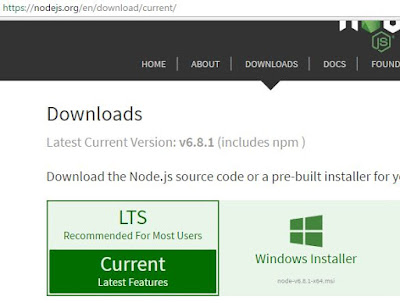
Post node.js installation, open "Node.js command prompt" from Start menu. Check the node and npm versions. Make sure you have these or later versions.
For npm version : npm -v
For node version : node -v
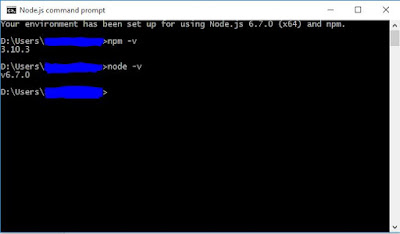
Run the following command to install native script package from Node.js command prompt
npm install -g nativescript
To verify the telerik nativescript installation, type "tns" and hit enter in the command prompt.
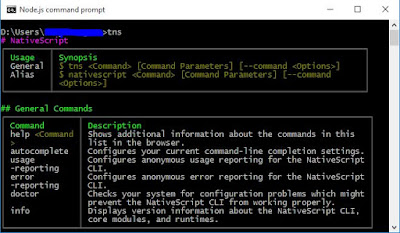
Go to the website - https://developer.android.com/studio/index.html
Scroll to the bottom.
We don't need to install the entire Android Studio, just click on the highlighted link to download the Android Software Development Kit. Install the SDK after download.
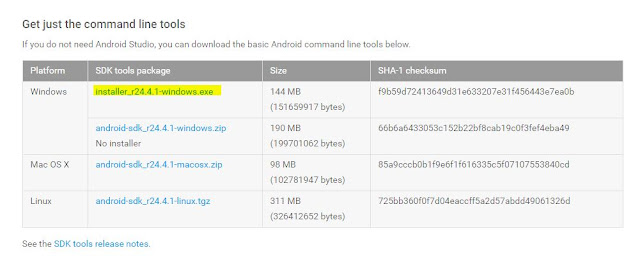
Now, you should be seeing two applications installed in "All Programs" menu from Start button.
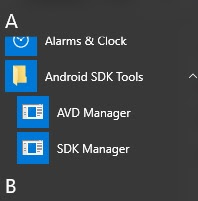
Right click SDK Manager and "Run as administrator".
Check three options under "Android 6.0 (API 23) and click "Install packages".
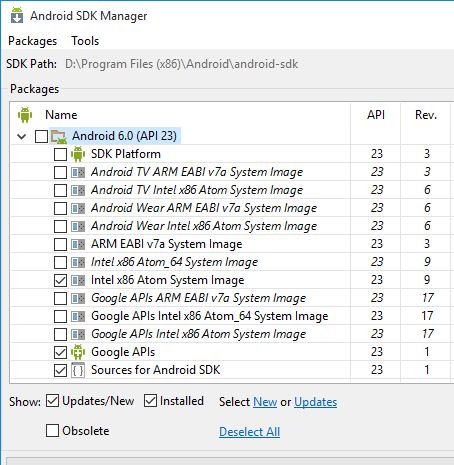
Also, Check four options under "Extras".
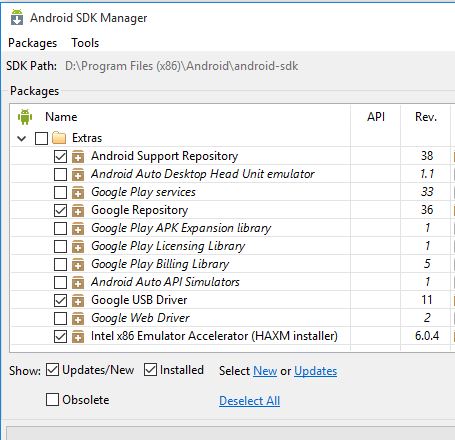
Right click on AVD (Android Virtual Device) manager from "Start Menu" and click "run as administrator".
In AVD Manager home screen, click "Create" and enter the exact following information. Click OK.
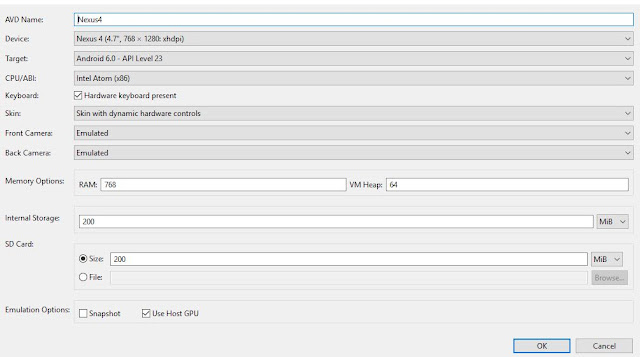
After this, you should be seeing this screen.
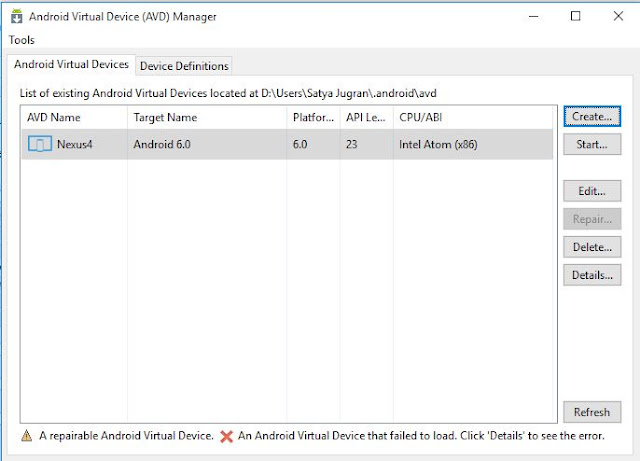
Go to the folder - "C:\Program Files (x86)\Android\android-sdk\extras\intel\Hardware_Accelerated_Execution_Manager"
Double click - "intelhaxm-android.exe" to execute the wizard and finish the set up.
Now in AVD Manager, click on "Start" on the right side options. The following screen should appear.
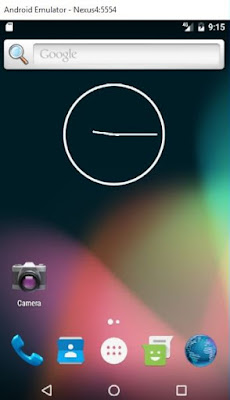
Open Command prompt from "Start" menu as an administrator and paste the following command and hit enter to execute.
@powershell -NoProfile -ExecutionPolicy Bypass -Command "iex ((new-object net.webclient).DownloadString('https://www.nativescript.org/setup/win'))"
We have seen that the documentation provided on internet is not the way it should be. This is because it includes four entirely different setups (Node, Android SDK, NativeScript and GIT). Issues occurs and we end up in wasting much time in internet search to fix those issue. We know the issues well and hence have tried to document this process from scratch where you have just....
>>>> Windows 10 installed on your machine. .... And Nothing Else
Please follow the mentioned steps in sequence (remember the only pre-requisite being Windows 10).
1) Install Node js version 6.7.0 or later.
You can download the same from -
https://nodejs.org/en/download/current
2) NodeJS version check.
Post node.js installation, open "Node.js command prompt" from Start menu. Check the node and npm versions. Make sure you have these or later versions.
For npm version : npm -v
For node version : node -v
3) Nativescript library for Nodejs installation.
Run the following command to install native script package from Node.js command prompt
npm install -g nativescript
4) Nativescript nodejs libriary installation verification.
To verify the telerik nativescript installation, type "tns" and hit enter in the command prompt.
5) Android SDK installation:
Go to the website - https://developer.android.com/studio/index.html
Scroll to the bottom.
We don't need to install the entire Android Studio, just click on the highlighted link to download the Android Software Development Kit. Install the SDK after download.
Now, you should be seeing two applications installed in "All Programs" menu from Start button.
6) Android packages installation.
Right click SDK Manager and "Run as administrator".
Check three options under "Android 6.0 (API 23) and click "Install packages".
Also, Check four options under "Extras".
7) Android Virtual Device configuration.
Right click on AVD (Android Virtual Device) manager from "Start Menu" and click "run as administrator".
In AVD Manager home screen, click "Create" and enter the exact following information. Click OK.
After this, you should be seeing this screen.
8) Andriod HAXM installation.
Go to the folder - "C:\Program Files (x86)\Android\android-sdk\extras\intel\Hardware_Accelerated_Execution_Manager"
Double click - "intelhaxm-android.exe" to execute the wizard and finish the set up.
9) Andriod Emulator preview.
Now in AVD Manager, click on "Start" on the right side options. The following screen should appear.
10) Nativescript for Windows installation.
Open Command prompt from "Start" menu as an administrator and paste the following command and hit enter to execute.
@powershell -NoProfile -ExecutionPolicy Bypass -Command "iex ((new-object net.webclient).DownloadString('https://www.nativescript.org/setup/win'))"
Verify the set up by executing the below command.
tns doctor
If you see "No issues were detected" you are good to go!
Go to the URL - https://git-scm.com/downloads
and click "Windows" under "Downloads" section. After download install the setup.
Create a folder in any location in your drive. (say test)
Now, from start menu - Open "Git Bash" as an administrator.
In Git bash, go to the location where you have created the "test" folder. Paste the following to Git clone a sample nativescript app.
git clone https://github.com/NativeScript/sample-Groceries.git
In Git bash, go to the repo folder
cd sample-Groceries
In Git bash, check out the version
git checkout angular-start
Open command prompt as an administrator and go to the "sample-Groceries" folder created in previous step. Paste the below command and hit enter to execute.
tns platform add android
now run the following command :
tns run android --emulator
You should be seeing the "Hello World" app hosted in the Android emulator.
You have successfully created and Angular 2 app in Android with NativeScript.
Note : Please post comments, in case you find any issues. Thanks!
tns doctor
If you see "No issues were detected" you are good to go!
11) GIT installation.
Go to the URL - https://git-scm.com/downloads
and click "Windows" under "Downloads" section. After download install the setup.
12) Nativescript's Angular 2 sample app setup.
Create a folder in any location in your drive. (say test)
Now, from start menu - Open "Git Bash" as an administrator.
In Git bash, go to the location where you have created the "test" folder. Paste the following to Git clone a sample nativescript app.
git clone https://github.com/NativeScript/sample-Groceries.git
In Git bash, go to the repo folder
cd sample-Groceries
In Git bash, check out the version
git checkout angular-start
11) Sample app execution in Andriod simulator.
Open command prompt as an administrator and go to the "sample-Groceries" folder created in previous step. Paste the below command and hit enter to execute.
tns platform add android
now run the following command :
tns run android --emulator
12) Congratulation!
You have successfully created and Angular 2 app in Android with NativeScript.
Note : Please post comments, in case you find any issues. Thanks!